클래스
class, self, 사용자 정의 데이터 타입, special methods(__init__(), __add_() ...)
변수, 함수를 묶어서 코드를 작성하는 방법
객체지향을 구현하는 문법
객체지향 : 실제세계를 모델링하여 프로그램을 개발하는 개발 방법론
=> 협업이 용이함
객체지향 참조
객체 지향 프로그래밍 - 위키백과, 우리 모두의 백과사전
위키백과, 우리 모두의 백과사전. 객체 지향 프로그래밍(영어: Object-Oriented Programming, OOP)은 컴퓨터 프로그래밍의 패러다임 중 하나이다. 객체 지향 프로그래밍은 컴퓨터 프로그램을 명령어의 목
ko.wikipedia.org
클래스 사용법
- 클래스선언(코드작성) => 객체생성(메모리사용) => 메서드실행(코드실행)
이것을 실제 세계에서...
- 클래스선언(설계도작성) => 객체생성(제품생산) => 메서드실행(기능사용)
로 비유할 수 있다!
메서드 : 클래스 안에서 선언된 함수
식별자 컨벤션
- 변수, 함수 : snake_case
- 클래스 : PascalCase(UpperCamelCase)
그럼 간단한 계산기 클래스를 작성해보자
1. 클래스 선언 : 코드작성
class Calculator:
number1, number2 = 1, 2
def plus(self):
return self.number1 + self.number2
def minus(self):
return self.number1 - self.number2
일단 덧셈, 뺄셈을 계산하는 클래스를 선언한다.
2. 객체생성 : 메모리사용
class Calculator:
number1, number2 = 1, 2
def plus(self):
return self.number1 + self.number2
def minus(self):
return self.number1 - self.number2
calc1 = Calculator()
calc2 = Calculator()
아래에 calc1, calc2 객체를 생성해줬다.
3. 메서드 실행 : 코드실행
class Calculator:
number1, number2 = 1, 2
def plus(self):
return self.number1 + self.number2
def minus(self):
return self.number1 - self.number2
calc1 = Calculator()
calc2 = Calculator()
calc1.plus(), calc1.minus()
메서드 실행하는 코드를 추가해줬고
결과는 아래와 같다.
1과 2만으로 계산을 할 수 없으니...
객체의 변수를 수정하고 싶으면...
데이터선택 = 수정할데이터의 형식으로 변수를 수정해준다!
class Calculator:
number1, number2 = 1, 2
def plus(self):
return self.number1 + self.number2
def minus(self):
return self.number1 - self.number2
calc1 = Calculator()
calc2 = Calculator()
calc1.plus(), calc1.minus()
print(calc1.number1, calc1.number2, calc2.number1, calc2.number2)
calc1.number1 = 20 # 객체의 변수 수정
print(calc1.number1, calc1.number2, calc2.number1, calc2.number2)
원래 객체의 변수는
calc1.number1 = 1, calc1.number2 = 2, calc2.number1 = 1, calc2.number2 = 2였지만
데이터를 수정 한 결과
calc1.number1 = 20, calc1.number2 = 2, calc2.number1 = 1, calc2.number2 = 2으로 바뀌었다!
class Calculator:
number1, number2 = 1, 2
def plus(self):
return self.number1 + self.number2
def minus(self):
return self.number1 - self.number2
calc1 = Calculator()
calc2 = Calculator()
calc1.plus(), calc1.minus()
print(calc1.number1, calc1.number2, calc2.number1, calc2.number2)
calc1.number1 = 20 # 객체의 변수 수정
print(calc1.number1, calc1.number2, calc2.number1, calc2.number2)
calc1.plus(), calc2.plus()
다시 각각 계산해 보면...
이 된다.
돈을 입금하고 인출하는 클래스를 보자!
# 클래스선언 : 은행계좌 : Account : balance, insert(), withdraw()
class Account :
balance = 0
def insert(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
print(f'잔액이 {amount-self.balance}원 부족합니다')
일단 먼제 클래스를 선언하고!
class Account :
balance = 0
def insert(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
print(f'잔액이 {amount-self.balance}원 부족합니다')
account_1 = Account()
account_2 = Account()
account_1.balance, account_2.balance
객체를 생성해 준다.
이렇게 account_1과 account_2 객체에 각각 계좌잔액이 0원, 0원 인것을 확인한다.
class Account :
balance = 0
def insert(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
print(f'잔액이 {amount-self.balance}원 부족합니다')
account_1 = Account()
account_2 = Account()
account_1.insert(10000)
account_1.withdraw(15000)
account_1.balance, account_2.balance
이렇게 account1에 10000원을 입금하고, account2에서 15000원을 출금하는 메서드를 실행하면...
아래와 같은 결과가 나온다.
만약 클래스 생성에서 변수의 초기값을 설정하지 않으면 어떻게 될까?
이렇게 변수의 초기값을 설정해주지 않은 클래스를 생성하고 객체도 생성한 뒤 메서드를 실행했다!
# 클래스 생성
class Account :
def insert(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
print(f'잔액이 {amount-self.balance}원 부족합니다')
account = Account() # 객체 생성
account.insert(3000) # 메서드 실행
위와 같은 오류가 나온다...
불량객체가 만들어져 메서드가 실행불가하다!
이러한 오류를 방지하기 위해 스페셜 메서드인 __init__()(생성자 메서드)를 사용한다.
스페셜 메서드 : 특별한 기능을 하는 메서드로 앞뒤로 __를 붙인다.
생성자 메서드
- __init__()
- 객체를 생성할때 실행되는 메서드
- 변수의 초기값을 설정할때 주로 사용
- 불량 객체(메서드 사용 X)가 만들어질 확률을 줄여줌
다시 코드로 돌아가서 아래와 같이 생성자 메서드를 써준다.
# 클래스 생성
class Account :
def __init__(self, balance):
self.balance = balance
def insert(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
print(f'잔액이 {amount-self.balance}원 부족합니다')
account = Account() # 객체 생성
account.insert(3000) # 메서드 실행
그럼 아래와 같은 오류가 나오는데...
객체가 생성될 때 account = Account()의 괄호 안에 예금을 넣지 않아서 발생하는 오류이다.
# 클래스 생성
class Account :
def __init__(self, balance):
self.balance = balance
def insert(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
print(f'잔액이 {amount-self.balance}원 부족합니다')
account = Account(10000) # 객체 생성
account.insert(3000) # 메서드 실행
account.balance
이렇게 객체를 생성할 때 10000원을 넣어주고 잔액을 확인해보면
3000원이 입금된
이 나온다.
클래스를 생성할 때 초기값을 넣어줄 수도 있다!
# 클래스 생성 : 설계도작성
class Account :
def __init__(self, balance=20000): # 초기값 넣어줄 수 있다.
self.balance = balance
def insert(self, amount):
self.balance += amount
def withdraw(self, amount):
if self.balance >= amount:
self.balance -= amount
else:
print(f'잔액이 {amount-self.balance}원 부족합니다')
account = Account()
account.insert(3000)
account.balance
위와 같이 __init__생성자의 파라미터에 초기값(여기서는 20000원)을 넣어주면
객체를 생성할때 괄호안에 따로 값을 넣어주지 않더라도 초기값으로 결과가 잘 나온다.
추가적으로...
클래스는 사용자 정의 데이터 타입이다!
위의 계좌클래스를 예로 들어서 살펴보자!
type(account)
이렇게 account객체의 데이터 타입을 확인해 보면
이렇게 사용자가 정의한 Account가 데이터 타입이다.
이번엔 account객체에서 사용가능한 메서드를 살펴보자!
객체에서 사용가능한 메서드를 출력하는 코드는 dir()함수이다.
dir(account)
이렇게 확인해 보면
뭐가 잔뜩나오는데... 앞뒤로 __가 들어간 메서드는 객체를 생성할때 자동으로 생성되는 것들이다.
[var for var in dir(account) if var[0] != '_']
__가 들어간 코드를 제거하고 우리가 생성한 메서드를 살펴보면
이렇게 3개의 메서드가 나온다.
이번 시간에는 클래스에 대해서 알아봤습니다...
진짜 클라스 지리네여...
막내집 재벌 포스납니다... ㅎㄷㄷ
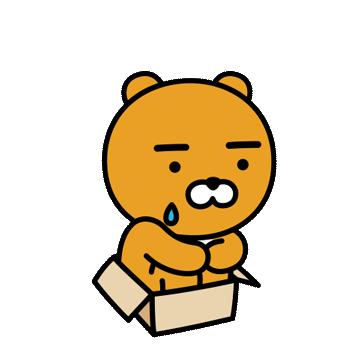
'멋쟁이사자처럼 AI스쿨' 카테고리의 다른 글
매출늘려버리기!(파이썬 강의 Day4) (0) | 2023.01.05 |
---|---|
상속과 데코레이터(파이썬 강의 Day4) (0) | 2023.01.05 |
함수3(파이썬 강의 Day3) (0) | 2023.01.04 |
함수2(파이썬 강의 Day3) (0) | 2023.01.04 |
함수1(파이썬 강의 Day3) (0) | 2023.01.04 |
댓글